Adds sparse updates to a variable reference.
This operation computes
# Scalar indices ref[indices, ...] += updates[...]
# Vector indices (for each i) ref[indices[i], ...] += updates[i, ...]
# High rank indices (for each i, ..., j) ref[indices[i, ..., j], ...] += updates[i, ..., j, ...]
This operation outputs `ref` after the update is done. This makes it easier to chain operations that need to use the reset value.
Duplicate entries are handled correctly: if multiple `indices` reference the same location, their contributions add.
Requires `updates.shape = indices.shape + ref.shape[1:]` or `updates.shape = []`.
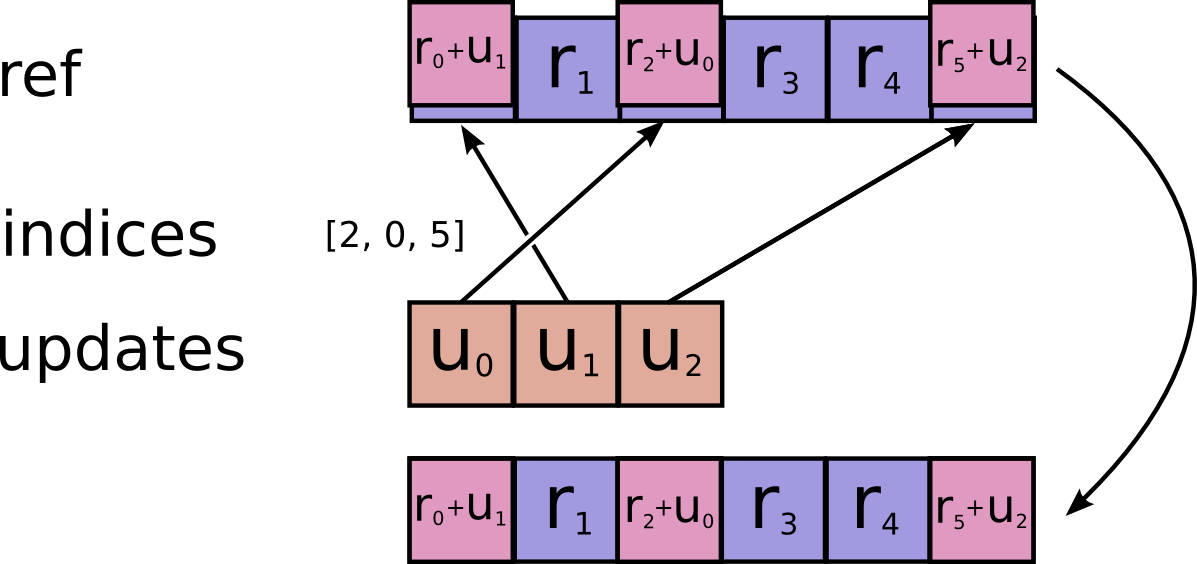
Nested Classes
class | ScatterAdd.Options | Optional attributes for ScatterAdd
|
Public Methods
Output<T> |
asOutput()
Returns the symbolic handle of a tensor.
|
static <T, U extends Number> ScatterAdd<T> | |
Output<T> |
outputRef()
= Same as `ref`.
|
static ScatterAdd.Options |
useLocking(Boolean useLocking)
|
Inherited Methods
Public Methods
public Output<T> asOutput ()
Returns the symbolic handle of a tensor.
Inputs to TensorFlow operations are outputs of another TensorFlow operation. This method is used to obtain a symbolic handle that represents the computation of the input.
public static ScatterAdd<T> create (Scope scope, Operand<T> ref, Operand<U> indices, Operand<T> updates, Options... options)
Factory method to create a class wrapping a new ScatterAdd operation.
Parameters
scope | current scope |
---|---|
ref | Should be from a `Variable` node. |
indices | A tensor of indices into the first dimension of `ref`. |
updates | A tensor of updated values to add to `ref`. |
options | carries optional attributes values |
Returns
- a new instance of ScatterAdd
public Output<T> outputRef ()
= Same as `ref`. Returned as a convenience for operations that want to use the updated values after the update is done.
public static ScatterAdd.Options useLocking (Boolean useLocking)
Parameters
useLocking | If True, the addition will be protected by a lock; otherwise the behavior is undefined, but may exhibit less contention. |
---|