Applies sparse updates to a variable reference.
This operation computes
# Scalar indices
ref[indices, ...] = updates[...]
# Vector indices (for each i)
ref[indices[i], ...] = updates[i, ...]
# High rank indices (for each i, ..., j)
ref[indices[i, ..., j], ...] = updates[i, ..., j, ...]
If values in `ref` is to be updated more than once, because there are duplicate entries in `indices`, the order at which the updates happen for each value is undefined.
Requires `updates.shape = indices.shape + ref.shape[1:]` or `updates.shape = []`.
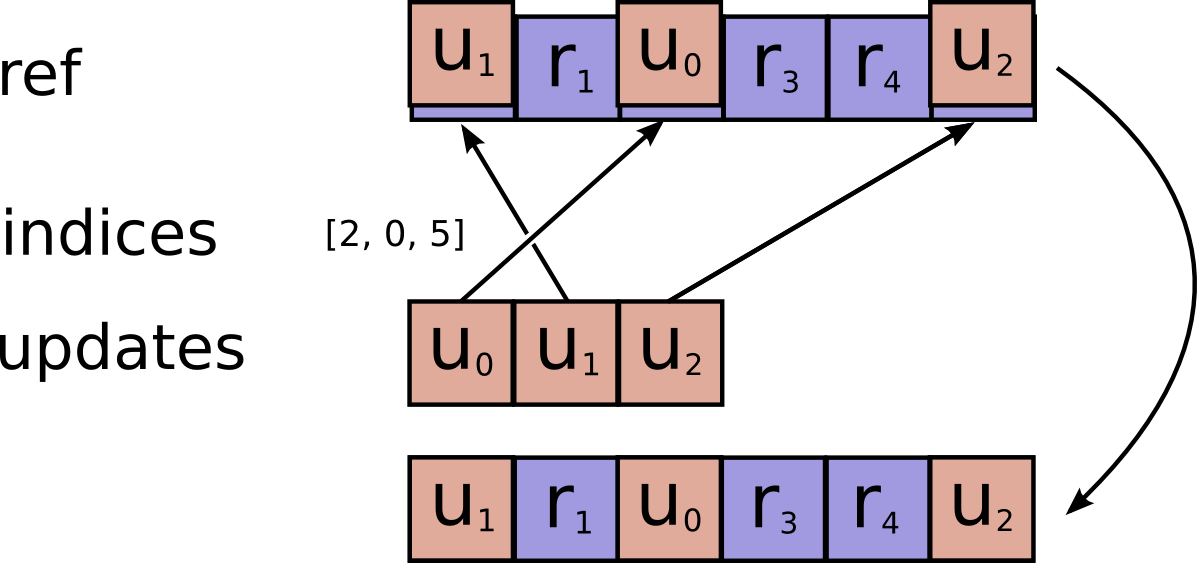
See also `tf.batch_scatter_update` and `tf.scatter_nd_update`.
Nested Classes
class | ScatterUpdate.Options | Optional attributes for ScatterUpdate
|
Public Methods
Output<T> |
asOutput()
Returns the symbolic handle of a tensor.
|
static <T, U extends Number> ScatterUpdate<T> | |
Output<T> |
outputRef()
= Same as `ref`.
|
static ScatterUpdate.Options |
useLocking(Boolean useLocking)
|
Inherited Methods
boolean |
equals(Object arg0)
|
final Class<?> |
getClass()
|
int |
hashCode()
|
final void |
notify()
|
final void |
notifyAll()
|
String |
toString()
|
final void |
wait(long arg0, int arg1)
|
final void |
wait(long arg0)
|
final void |
wait()
|
Public Methods
public Output<T> asOutput ()
Returns the symbolic handle of a tensor.
Inputs to TensorFlow operations are outputs of another TensorFlow operation. This method is used to obtain a symbolic handle that represents the computation of the input.
public static ScatterUpdate<T> create (Scope scope, Operand<T> ref, Operand<U> indices, Operand<T> updates, Options... options)
Factory method to create a class wrapping a new ScatterUpdate operation.
Parameters
scope | current scope |
---|---|
ref | Should be from a `Variable` node. |
indices | A tensor of indices into the first dimension of `ref`. |
updates | A tensor of updated values to store in `ref`. |
options | carries optional attributes values |
Returns
- a new instance of ScatterUpdate
public Output<T> outputRef ()
= Same as `ref`. Returned as a convenience for operations that want to use the updated values after the update is done.
public static ScatterUpdate.Options useLocking (Boolean useLocking)
Parameters
useLocking | If True, the assignment will be protected by a lock; otherwise the behavior is undefined, but may exhibit less contention. |
---|